mirror of
https://github.com/ghostty-org/ghostty.git
synced 2025-04-23 18:08:39 +03:00
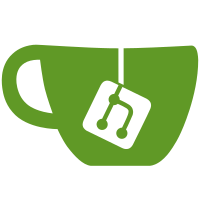
Related to #5361 The fix in 5361 wasn't sufficient since it only applied if our app was in the foreground. Our quick terminal is a non-activating NSPanel to allow it to work on any space (fullscreen included). This means that Ghostty doesn't become the active app when the quick terminal is shown and another app is in the foreground. To work around this, we now hide the dock globally when the quick terminal is shown AND the dock is in a conflicting position. We restore this state when the quick terminal is hidden, loses focus, or Ghostty is quit.
39 lines
1.1 KiB
Swift
39 lines
1.1 KiB
Swift
import Cocoa
|
|
|
|
// Private API to get Dock location
|
|
@_silgen_name("CoreDockGetOrientationAndPinning")
|
|
func CoreDockGetOrientationAndPinning(
|
|
_ outOrientation: UnsafeMutablePointer<Int32>,
|
|
_ outPinning: UnsafeMutablePointer<Int32>)
|
|
|
|
// Private API to get the current Dock auto-hide state
|
|
@_silgen_name("CoreDockGetAutoHideEnabled")
|
|
func CoreDockGetAutoHideEnabled() -> Bool
|
|
|
|
// Toggles the Dock's auto-hide state
|
|
@_silgen_name("CoreDockSetAutoHideEnabled")
|
|
func CoreDockSetAutoHideEnabled(_ flag: Bool)
|
|
|
|
enum DockOrientation: Int {
|
|
case top = 1
|
|
case bottom = 2
|
|
case left = 3
|
|
case right = 4
|
|
}
|
|
|
|
class Dock {
|
|
/// Returns the orientation of the dock or nil if it can't be determined.
|
|
static var orientation: DockOrientation? {
|
|
var orientation: Int32 = 0
|
|
var pinning: Int32 = 0
|
|
CoreDockGetOrientationAndPinning(&orientation, &pinning)
|
|
return .init(rawValue: Int(orientation)) ?? nil
|
|
}
|
|
|
|
/// Set the dock autohide.
|
|
static var autoHideEnabled: Bool {
|
|
get { return CoreDockGetAutoHideEnabled() }
|
|
set { CoreDockSetAutoHideEnabled(newValue) }
|
|
}
|
|
}
|