mirror of
https://github.com/ghostty-org/ghostty.git
synced 2025-07-04 05:08:39 +03:00
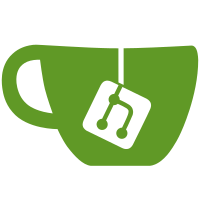
* update zig * pkg/fontconfig: clean up @as * pkg/freetype,harfbuzz: clean up @as * pkg/imgui: clean up @as * pkg/macos: clean up @as * pkg/pixman,utf8proc: clean up @as * clean up @as * lots more @as cleanup * undo flatpak changes * clean up @as
89 lines
2.5 KiB
Zig
89 lines
2.5 KiB
Zig
const std = @import("std");
|
|
const assert = std.debug.assert;
|
|
const Allocator = std.mem.Allocator;
|
|
const foundation = @import("../foundation.zig");
|
|
const text = @import("../text.zig");
|
|
const c = @import("c.zig");
|
|
|
|
pub const AttributedString = opaque {
|
|
pub fn release(self: *AttributedString) void {
|
|
foundation.CFRelease(self);
|
|
}
|
|
|
|
pub fn getLength(self: *AttributedString) usize {
|
|
return @intCast(c.CFAttributedStringGetLength(@ptrCast(self)));
|
|
}
|
|
|
|
pub fn getString(self: *AttributedString) *foundation.String {
|
|
return @ptrFromInt(@intFromPtr(
|
|
c.CFAttributedStringGetString(@ptrCast(self)),
|
|
));
|
|
}
|
|
};
|
|
|
|
pub const MutableAttributedString = opaque {
|
|
pub fn create(cap: usize) Allocator.Error!*MutableAttributedString {
|
|
return @as(
|
|
?*MutableAttributedString,
|
|
@ptrFromInt(@intFromPtr(c.CFAttributedStringCreateMutable(
|
|
null,
|
|
@intCast(cap),
|
|
))),
|
|
) orelse Allocator.Error.OutOfMemory;
|
|
}
|
|
|
|
pub fn release(self: *MutableAttributedString) void {
|
|
foundation.CFRelease(self);
|
|
}
|
|
|
|
pub fn replaceString(
|
|
self: *MutableAttributedString,
|
|
range: foundation.Range,
|
|
replacement: *foundation.String,
|
|
) void {
|
|
c.CFAttributedStringReplaceString(
|
|
@ptrCast(self),
|
|
range.cval(),
|
|
@ptrCast(replacement),
|
|
);
|
|
}
|
|
|
|
pub fn setAttribute(
|
|
self: *MutableAttributedString,
|
|
range: foundation.Range,
|
|
key: anytype,
|
|
value: ?*anyopaque,
|
|
) void {
|
|
const T = @TypeOf(key);
|
|
const info = @typeInfo(T);
|
|
const Key = if (info != .Pointer) T else info.Pointer.child;
|
|
const key_arg = if (@hasDecl(Key, "key"))
|
|
key.key()
|
|
else
|
|
key;
|
|
|
|
c.CFAttributedStringSetAttribute(
|
|
@ptrCast(self),
|
|
range.cval(),
|
|
@ptrCast(key_arg),
|
|
value,
|
|
);
|
|
}
|
|
};
|
|
|
|
test "mutable attributed string" {
|
|
//const testing = std.testing;
|
|
|
|
const str = try MutableAttributedString.create(0);
|
|
defer str.release();
|
|
|
|
{
|
|
const rep = try foundation.String.createWithBytes("hello", .utf8, false);
|
|
defer rep.release();
|
|
str.replaceString(foundation.Range.init(0, 0), rep);
|
|
}
|
|
|
|
str.setAttribute(foundation.Range.init(0, 0), text.FontAttribute.url, null);
|
|
str.setAttribute(foundation.Range.init(0, 0), text.FontAttribute.name.key(), null);
|
|
}
|